새싹교실/2014/다빈치인재반/11회차 (rev. 1.13)
- Associative array
- Maps in STL
* JSON
- Binary Search Tree
- Hash Table(간략하게, Tree 위주. Associative array를 설명할 때 필요는 하므로.)
3.1. Associative array ¶
- In computer science, an associative array, map, symbol table, or dictionary is an abstract data type composed of a collection of (key, value) pairs, such that each possible key appears at most once in the collection.
- In an associative array, the association between a key and a value is often known as a "binding", and the same word "binding" may also be used to refer to the process of creating a new association.
- Add or insert: add a new (key, value) pair to the collection, binding the new key to its new value. The arguments to this operation are the key and the value.
- Reassign: replace the value in one of the (key, value) pairs that are already in the collection, binding an old key to a new value. As with an insertion, the arguments to this operation are the key and the value.
- Remove or delete: remove a (key, value) pair from the collection, unbinding a given key from its value. The argument to this operation is the key.
- Lookup: find the value (if any) that is bound to a given key. The argument to this operation is the key, and the value is returned from the operation. If no value is found, some associative array implementations raise an exception.
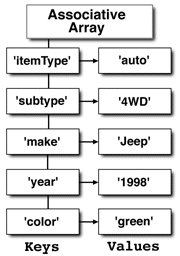
[GIF image (9.21 KB)]
#include<iostream>
#include<string>
#include<map>
using namespace std;
void printCarInfo(map <string,string> &carinfo){
cout<<"----------------------------------------------"<<endl;
map <string, string>::iterator it1;
for(it1 = carinfo.begin(); it1 != carinfo.end(); it1++){
cout<<it1->first<<" : "<<it1->second<<endl;
}
}
int main(void){
map <string, string> carinfo;
carinfo.insert(map<string, string>::value_type("itemType", "auto"));
carinfo.insert(make_pair("subtype", "4WD"));
carinfo["make"] = "Jeep";
carinfo["year"] = "1998";
carinfo["color"] = "green";
//Insert Key-Value Pair
printCarInfo(carinfo);
map <string, string>::iterator FindIter = carinfo.find("year");
if(FindIter != carinfo.end()){
FindIter->second = "2002";
}
//Lookup & Reassign
printCarInfo(carinfo);
carinfo["year"] = "2013";
//Reassign
printCarInfo(carinfo);
carinfo.erase("year");
//delete
printCarInfo(carinfo);
}